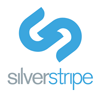
SilverStripe is an excellent, user-friendly content management system but its internal search functionality is, to put it kindly, useless. Fortunately with Google Site Search you can embed a Google-powered custom search engine into your SilverStripe site. Doing so requires a paid Site Search account, pricing for which starts at $100/year.
This tutorial explains how to integrate this Google Site Search XML feed into your SilverStripe site. Doing so has a number of benefits over the standard means of integrating Site Search, namely:
- No Javascript is required to display results within the SilverStripe site.
- The user is not taken to a separate, Google operated website to view results.
- The look and feel is consistent with the rest of the SilverStripe site.
- Multiple Site Search engines can be integrated into a single SilverStripe site.
- Site Search results pages are integrated into SilverStripe's management console.
Note: To integrate Site Search into SilverStripe using the described method a Site Search plan must be purchased as this provides results in XML. The free, advertising supported, Site Search engine does not provide search results in XML and cannot be used.
Loading XML data from an external source
Before the search page can be added to SilverStripe we need a reliable means of loading XML content. This is complicated by the fact many Web hosts disable PHP's built in URL fetcher (fopen) with the following php.ini directive:
allow_url_fopen = Off
Assuming it is installed, the cURL can get around this restriction, hence the XmlLoader helper library includes both methods (cURL is used by default in search.php).
Create a XmlLoader.php file in your SilverStripe's mysite/code directory with the following contents:
mysite/code/XmlLoader.php
<?php
class XmlLoader {
public function pullXml($url, $parameters, $useCurl) {
$urlString = $url."?".$this->buildParamString($parameters);
if ($useCurl) {
return simplexml_load_string($this->loadCurlData($urlString));
} else {
return simplexml_load_file($urlString);
}
}
private function loadCurlData($urlString) {
if ($urlString == -1) {
echo "No url supplied<br/>"."/n";
return(-1);
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $urlString);
curl_setopt($ch, CURLOPT_TIMEOUT, 180);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$data = curl_exec($ch);
curl_close($ch);
return $data;
}
private function buildParamString($parameters) {
$urlString = "";
foreach ($parameters as $key => $value) {
$urlString .= urlencode($key)."=".urlencode($value)."&";
}
if (trim($urlString) != "") {
$urlString = preg_replace("/&$/", "", $urlString);
return $urlString;
} else {
return (-1);
}
}
}
?>
With the helper library in place to load the XML, it is now time to implement the SilverStripe "search" page type and logic. Create a search.php file in your SilverStripe's mysite/code directory with the following contents:
mysite/code/search.php
<?php
require_once 'XmlLoader.php';
class Search extends Page {
static $db = array(
'GoogleSearchId' => 'Text',
'NoResults' => 'HTMLText',
);
static $has_one = array(
);
function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab('Root.Content.Main', new TextField(
'GoogleSearchId', 'Google Site Search ID'), 'Content');
$fields->addFieldToTab('Root.Content.Main', new HtmlEditorField(
'NoResults', 'No results message'), 'Content');
# Remove the content field
$fields->removeFieldFromTab("Root.Content.Main","Content");
return $fields;
}
}
class Search_Controller extends Page_Controller {
function SearchForm() {
$input = array_merge($_GET, $_POST);
$query = $input['q'];
$output = "<form class=\"search\" action=\"/search/results\"><fieldset>";
$output .= "<input type=\"text\" size=\"40\" name=\"q\" value=\"$query\"/>";
$output .= "<input type=\"hidden\" name=\"p\" value=\"1\"/>";
$output .= "<input type=\"submit\" value=\"Search\"/>";
$output .= "</fieldset></form>";
return $output;
}
function SearchResults() {
$output = "";
$input = array_merge($_GET, $_POST);
$page = isset($input['p']) ? $input['p'] : '1';
$query = $input['q'];
$perPage = 10;
if ($page < 1) { $page = 1; }
$xml = $this->getGoogleSearchResults($this->GoogleSearchId, $perPage, $page, $query);
$results = $this->parseGoogleSearchResults($xml);
$totalResults = $this->getResultCount($xml);
$output .= $this->getFormattedResults($results);
if (count($results) == 0) {
// Show no results message
$output .= $this->NoResults;;
} else {
// Append paging
$output .= $this->getPagingForResults($totalResults, $query, $perPage, $page);
}
return $output;
}
private function getGoogleSearchResults($googleId, $perPage, $page, $query) {
$startingRecord = ($page - 1) * $perPage;
$url = "http://www.google.com/search";
$parameters = array();
$parameters["client"] = "google-csbe";
$parameters["output"] = "xml_no_dtd";
$parameters["num"] = $perPage;
$parameters["cx"] = $googleId;
$parameters["start"] = $startingRecord;
$parameters["q"] = $query;
$XmlLoader = new XmlLoader();
return $XmlLoader->pullXml($url, $parameters, true);
}
private function parseGoogleSearchResults($xml) {
$results = array();
$attr["title"] = $xml->xpath("/GSP/RES/R/T");
$attr["url"] = $xml->xpath("/GSP/RES/R/U");
$attr["desc"] = $xml->xpath("/GSP/RES/R/S");
foreach($attr as $key => $attribute) {
$i = 0;
foreach($attribute as $element) {
$results[$i][$key] = (string)$element;
$i++;
}
}
return $results;
}
private function getFormattedResults($results) {
$output = "";
if (count($results) > 0) {
$output .= "<ul class=\"results\">";
foreach($results as $i => $result) {
$title = "";
$url = "";
$desc = "";
foreach($result as $key => $value) {
if ($key == "title") {
$title = $value;
}
if ($key == "url") {
$url = $value;
}
if ($key == "desc") {
$desc = $value;
}
$output .= "<li><a href=\"$url\">$title</a><p>";
$output .= str_replace("<br>", "<br/>", $desc);
$output .= "</p></li>\n";
}
$output .= "</ul>";
}
return $output;
}
private function getResultCount($xml) {
$totalResults = 0;
$count = $xml->xpath("/GSP/RES/M");
foreach($count as $value) {
$totalResults = $value;
}
return $totalResults;
}
private function getPagingForResults($totalResults, $query, $perPage, $page) {
$maxPage = ceil($totalResults/$perPage);
if ($totalResults > 1) {
$output = "<div class=\"searchPaging\"><p>";
for($pageNum = 1; $pageNum <= $maxPage; $pageNum++) {
if ($pageNum == $page) {
$output .= " <strong>$pageNum</strong> ";
} else {
$output .= " <a href=\"".$this->AbsoluteLink()."results?q=$query&p=$pageNum\">$pageNum</a> ";
}
}
$output .= "</p></div>";
}
return $output;
}
}
?>
This file defines a search page type with two fields, a Google Search Id and an HTML field that is displayed if no search results are found. As this page does not have any content of its own the default SilverStripe content field is also disabled to avoid confusion.
With the backend logic in place it is time to implement the templates. The templates themselves will vary from site to site, but the examples given are good starting points. There are two templates, one which simply displays the search box and a second that displays the results.
Create a Search.ss file in your SilverStripe's mysite/templates/Layout directory with the following contents:
mysite/templates/Layout/Search.ss
<% if Menu(2) %>
<div class="pageWithMenu">
<% end_if %>
<div class="page">
<% if Menu(2) %>
<div class="content contentStandard">
<% else %>
<div class="content contentFull">
<% end_if %>
<h1>$Title</h1>
<div class="contentWrapper">
$SearchForm
<div class="clear"><!-- --></div>
</div>
</div>
<% if Menu(2) %>
<div id="sidepanel">
<% include SideBar %>
</div>
<div class="clear"><!-- --></div>
</div>
<% end_if %>
Now create the results page named Search_results.ss in your SilverStripe's mysite/templates/Layout directory with the following contents:
mysite/templates/Layout/Search_results.ss
<% if Menu(2) %>
<div class="pageWithMenu">
<% end_if %>
<div class="page">
<% if Menu(2) %>
<div class="content contentStandard">
<% else %>
<div class="content contentFull">
<% end_if %>
<h1>$Title</h1>
<div class="contentWrapper">
$SearchForm
<div id="searchResults">
$SearchResults
</div>
</div>
<div class="clear"><!-- --></div>
</div>
</div>
<% if Menu(2) %>
<div id="sidepanel">
<% include SideBar %>
</div>
<div class="clear"><!-- --></div>
</div>
<% end_if %>
Note: The content of these two files will vary depending on your site. In the above example a SideBar include file is used to load the secondary menu.
With the backend logic and template files in place it is time to rebuild the SilverStripe database so that the new page type can be recognised. Enter the following (modified) URL into your browser: http://yourwebsite/dev/build?flush=all
All going well the rebuild command will execute correctly. If it does browse to the administration section of your site and create a 'search' page type.
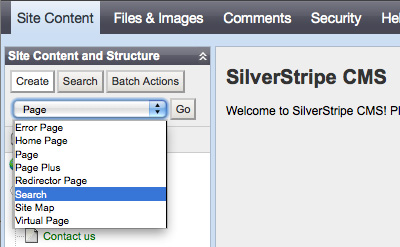
The search page type in the create menu
On the search page enter your relevant Google Search Id and Results Not Found message. For the page URL use /search as this is hard coded into the search.php file. It is possible to change this URL (or use a dynamic one) but for the purposes of this tutorial it is not necessary.
Note: You can get your Google Search Id from the Google Search administration console, or it can be found within the embed URL used in the Javascript or external search forms.
Once published open the page and try a search. Assuming your code and settings are correct the Google search results should be displayed within your SilverStripe page. Now all there is left for you to do is style the results.
For an example of this technique at work, checkout the Parliamentary Counsel Office's search interface which is implemented using the method just described.